C .
ODE
G
AMELET
person_outline
Sign In
videogame_asset
Public Games
local_library
Public Library
work
Public Projects
comment
Discuss
search
visibility
code
OPEN
info_outline
# 五十音 ~~BINGO~~ 這是一個用來練習五十音的小遊戲。 可以在主頁面設定要以平假名或是片假名為主。 開始後會產生 5 x 5 的隨機假名表,並且隨機產生對應的題目,如一開始選擇平假名,則題目將為片假名,根據題目選擇對應的假名即可圈選。 快來測試看看自己的速度如何吧! ~~花了一個晚上做的,原本要做 BINGO,但連線之後有機會再說吧~~~ ## Authors **[cook1470](/profile/cook1470)** ## 素材 ### Sprite - [basic-gui-bundle](https://penzilla.itch.io/basic-gui-bundle) - [Bing image creator](https://www.bing.com/images/create) ### Music - [Bossa Nova](https://opengameart.org/content/bossa-nova) ### Sound Effects - [Haskasu](https://code.gamelet.com/profile/Haskasu/assets) - [cook1470](https://code.gamelet.com/profile/cook1470/assets)
GojuuonBingo
cook1470
visibility
code
OPEN
info_outline
# 我的光暈戰記 My Twilight Wars 皇家近武能力測試 The template that help developers to build their own twilightwars-like game, your own map, your own mission. ## 快捷鈕 Shortcuts <a href="cg://source/CG.TWEventsGameTemplate/game.events" class="mat-raised-button mat-primary">編輯任務事件表 (Edit Events)</a> <a href="cg://source/CG.TWEventsGameTemplate/gamemap.twmap" class="mat-raised-button mat-primary">編輯地圖 (Edit Map)</a> ## 多重任務列表 將 appSeasons.ts 設為遊戲進入點,即可支援任務章節/列表。 <a href="cg://source/CG.TWEventsGameTemplate/seasons.ts" class="mat-raised-button mat-primary">編輯 seasons.ts 任務列表</a> Set appSeasons.ts as the entry point to support seasons/mission list. <a href="cg://source/CG.TWEventsGameTemplate/seasons.ts" class="mat-raised-button mat-primary">Edit seasons.ts (Mission List)</a>
皇家近武能力測試
36984
visibility
code
OPEN
info_outline
# MarutsukiMaruhiTenkiHaru 在這兒寫下一段簡短的文字介紹你的遊戲吧。 ## 開始設計 <a href="cg://source/CG.MarutsukiMaruhiTenkiHaru/game.events" class="mat-raised-button mat-primary">編輯遊戲事件表</a> ## 作者 **[Aya55](/profile/106797148482283198640@google)**
MarutsukiMaruhiTenkiHaru
Aya55
visibility
code
OPEN
info_outline
# Retry One Paragraph of the game description goes here ## Getting Started <a href="cg://source/CG.Retry/game.events" class="mat-raised-button mat-primary">Edit Game Events Sheet</a> ## Authors **[Ivan.Kong](/profile/106602187661106560490@google)**
突圍而出
Ivan.Kong
visibility
code
OPEN
info_outline
# PracticeZombieUI 這是一個用於模擬顯示光暈戰記 - 殭屍來襲中,商店 UI 的事件表模組。 ### 包含模組 - [TwilightWarsLib](https://twilightwarslib.gamelet.online/) ## 使用介紹 1. 在想要啟用 UI 的事件表中,用於初始化的事件中**添加兩個全域變數**,用於代表**天數**、**金幣**。 2. 宣告變數之後再添加**「殭屍來襲 UI:初始化」**動作,並代數第 1 步新增的兩個全域變數。 3. 可使用動作**「殭屍來襲 UI:添加商品」**添加商品,若須自訂圖示則需使用自訂武器道具。 4. 若要改變天數、金幣請使用動作**「殭屍來襲 UI:設置天數」**、**「殭屍來襲 UI:設置金幣」**。 5. 可自行接收觸發**「殭屍來襲 UI:選擇商品」**來實踐選擇商品後的事件動作。<br/>*觸發的選擇商品只有在玩家選擇商品,且金幣可購買商品時觸發。* ## Authors **[cook1470](/profile/cook1470)**
光暈戰記:殭屍來襲 UI
cook1470
visibility
code
OPEN
info_outline
# 畫線畫圖演算法 - 布雷森漢姆直線演算法 (Bresenham's line algorithm) - 吳小林直線演算法 (Xiaolin Wu's line algorithm) - 中點畫圓演算法 (Midpoint Circle algorithm) - 反鋸齒畫圓演算法 (Antialias Circle algorithm) # 影片講解 [畫線演算法] https://www.youtube.com/watch?v=YAsKbVYS6jY [畫圓演算法] https://www.youtube.com/watch?v=dMyCjrYCD2w
Line Renderer
Haskasu
visibility
code
OPEN
info_outline
# 為啥老在for迴圈裏寫 ++i (而不是 i++) 影片在這啦 - 《隨口講講系列:為什麼喜歡在for迴圈裏寫 ++i》 https://www.youtube.com/watch?v=P44ly_VOjF0 ## Authors **[Haskasu](/profile/Haskasu)**
iplusplus
Haskasu
visibility
code
OPEN
info_outline
Arcana
Rekka
visibility
code
OPEN
info_outline
九種職業 (冒險者 薩滿 偵探 遊俠 忍者 卜筮 惡魔 沙漠盜賊 冰原行者) 四段加強(每五依序解鎖 吸血100% 增傷一倍 10%護盾 每5次爆擊 且每個職業主要輸出每等1.05倍 血量+20%) 隨機生怪 擊敗首領(殺死25隻任意怪物 即會召喚地下城之主 50%護盾 集氣四方衝擊波回到定點再召喚一次衝擊波)
勇闖地下城
Ryuyan
visibility
code
OPEN
info_outline
# VNtest 在這兒寫下一段簡短的文字介紹你的遊戲吧。 ## 開始設計 <a href="cg://source/CG.VNtest/game.events" class="mat-raised-button mat-primary">編輯遊戲事件表</a> ## 作者 **[F1q6Y](/profile/104004080943013842966@google)**
NCUday
WagashiFish
visibility
code
OPEN
info_outline
# test_threejs One Paragraph of project description goes here ## Getting Started (For a game project) Write some tips or instructions how to control in your game. (For building a module) Write a piece of codes to demostrate how to use this module. ```typescript function examples() { } ``` ## Authors **[Haskasu](/profile/Haskasu)**
test_threejs
Haskasu
visibility
code
OPEN
info_outline
# three.js three.js 是一個基於 JavaScript 的瀏覽器 3D 繪圖引擎,允許開發者在網頁上輕鬆創建和顯示 3D 場景。 ## 使用方法 以下是一個簡單的範例,展示如何使用 `three.js` 創建一個基本的 3D 場景: ```ts import three = CG.ThreeJs.three; import OrbitControls = CG.ThreeJs.examples.jsm.controls.OrbitControls; function start(): void { // 初始化 three,用於自動建立場景、攝影機、渲染循環。 three.initialize(800, 600); // 建立一個軸心輔助物件,用於查看 x, y, z 的方向 const axesHelper = new THREE.AxesHelper(2); three.scene.add(axesHelper); // 添加至場景中 // 創建一個平面作為地板 const planeGeometry = new THREE.PlaneGeometry(10, 10); // 表示平面的幾何形狀 const planeMaterial = new THREE.MeshBasicMaterial({ color: 0xAAAAAA, side: THREE.DoubleSide }); // 基本紋理 const plane = new THREE.Mesh(planeGeometry, planeMaterial); plane.rotation.x = Math.PI / 2; // 平面一開始為垂直,調整 X 軸旋轉角度使其水平 three.scene.add(plane); // 將平面添加至場景中 // 創建方塊 const boxGeometry = new THREE.BoxGeometry(0.5, 0.5, 0.5); // 表示立方體的幾何形狀 const noxMaterial = new THREE.MeshBasicMaterial({ color: 0x00ff00 }); // 基本紋理 const box = new THREE.Mesh(boxGeometry, noxMaterial); box.position.y = 0.25; // 將方塊抬高 0.25,使其貼地 three.scene.add(box); // 將方塊添加至場景中 three.camera.position.set(0, 2, 5); // 調整攝影機位置 three.camera.lookAt(0, 0, 0); // 讓攝影機看向 (0, 0, 0) // 本模組初始化時會自動建立攝影機,一般需自行建立 // 新增一個控制器,可以用滑鼠來控制攝影機的視角,像是自由旋轉、平移和縮放效果。 const controls = new OrbitControls(three.camera, three.renderer.domElement); let lastFrame = Date.now(); three.beforeRender = () => { // 你可以複寫這個函數,在渲染畫面前做點事 const currentFrame = Date.now(); // 為當前幀時間 const deltaTime = currentFrame - lastFrame; // 計算自上幀以來的時間差 lastFrame = currentFrame; // 更新 lastFrame 為當前幀時間 // 如果 controls.enableDamping 或 controls.autoRotate 設為 true 則必須每次渲染畫面前更新。 controls.update(deltaTime); // 更新 OrbitControls }; } start(); ``` ## Resources - [three.js](https://threejs.org/) - 基於 JavaScript 的瀏覽器 3D 繪圖引擎。 ## Authors **[cook1470](/profile/cook1470)**
ThreeJs
cook1470
visibility
code
OPEN
info_outline
# PixiGifMattKarl One Paragraph of project description goes here ## Getting Started (For a game project) Write some tips or instructions how to control in your game. (For building a module) Write a piece of codes to demostrate how to use this module. ```typescript function examples() { } ``` ## Authors **[cook1470](/profile/cook1470)**
PixiGifMattKarl
cook1470
visibility
code
OPEN
info_outline
# TwilightWarsParkourLib 主要目標是復刻舊版跑酷,順帶提供一些跑酷相關的功能。 註:全憑印象調整參數,與舊版不完全一樣。 而關於事件表如何使用,可參照demo資料夾。 ## 建議的跳躍按鍵參數 | | | | :----- | ----: | | 最慢跳躍速度百分比 | 70% | | 最快跳躍速度百分比 | 100% | | 向上跳躍力 | 50% | ## 已復刻的特性 - 原地助跑 - 切換武器影響連跳 - 斜角可穿越 - 舊版角跳 ## 事件表功能 (v0.0.1) - 光暈戰記 - 跑酷 - 套用舊版跑酷 - 設定可走格 - 設定地圖格子 - 篩選地圖格子 ## Getting Started ```typescript Parkour.activateForActor(actor); // 將該角色的跑酷設定更改為舊版 const jumpData = actor.activateJumpKey(); // 啟用按鍵跳躍 jumpData.speedScale.reset(0.7, 1); // 更改最慢跳躍速度百分比、最快跳躍速度百分比 jumpData.initVz = 0.5; // 更改向上跳躍力 if (actor.isMe()) { let myController = actor.controller as MyActorController; myController.assignJumpKey(CG.Base.keyboard.Key.SPACE); // 更改跳躍按鍵 } ``` ## 測試影片 [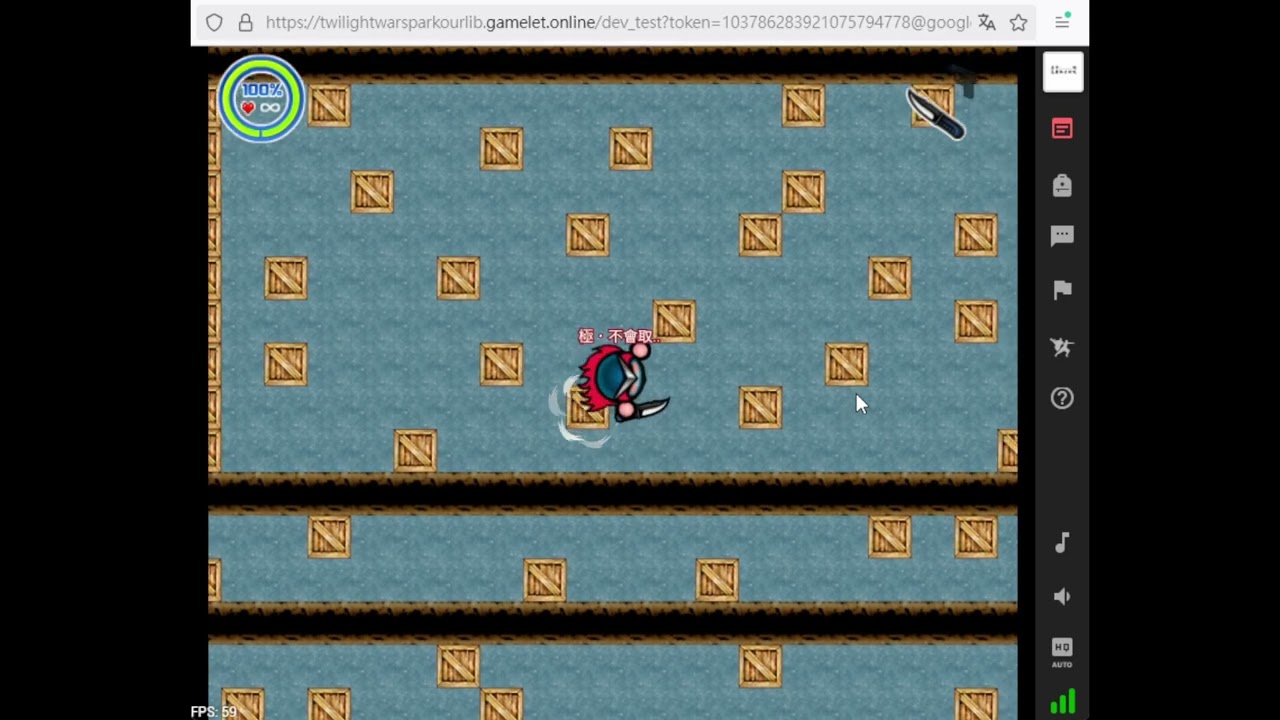](https://youtu.be/UkJASsSlq_Y "測試影片1") ## 作者 **[不會取名字](/profile/buhuechuminzu)**<br> **[雪姬](/profile/setsuki)**
TwilightWarsParkourLib
EnhProject
visibility
code
OPEN
info_outline
# 穆的光暈同人陣任務 Muk's Mission 光暈戰記的同人陣。 作者於新同人陣仍停留在學習階段,有bug/改進建議歡迎提出。 作者空閒時間不多,更新多於週末/假日出現。 目前進度: 皇家:第一部第一章結束 天影:第一部第一章第二節 第三:
MUK01
Mukmuk
visibility
code
OPEN
info_outline
# 光如繁性 我們是誰!勘查生!我們的任務是什麼!尋找說謊的人!! ## 開始設計 <a href="cg://source/CG.Light_as_complex/game.events" class="mat-raised-button mat-primary">編輯遊戲事件表</a> ## 作者 **[偷懶不香嗎](/profile/117559094754712190291@google)**
光如繁性
p6W1e
visibility
code
OPEN
info_outline
# 我的視覺小說(My Visual Novel) 視覺小說遊戲的範本專案,編輯事件表就可以做出自己的視覺小說遊戲了。 - [視覺小說製作教學影片](https://www.youtube.com/live/s19SacUQ0V4?si=4j4W3RXfyPj9bNrz&t=2562) <a href="https://code.gamelet.com/edit/VisualNovelGame?source=CG.VisualNovelGame/game.events" class="mat-raised-button mat-primary">點此參考範本專案</a> ### 開始設計 <a href="cg://source/CG.VisualNovelGameTemplate/game.events" class="mat-raised-button mat-primary">點此開始創造視覺小說遊戲</a> ## 如何複製這個專案? 1. 開啟本專案。  2. 點擊專案代碼右側的 🍴(刀叉圖示)來複製成新的專案。  3. 輸入你自己的專案代碼後,點摳成新專案。  # 專案介紹  本專案檔案總管如上圖所示,**視覺小說遊戲的主要流程在 `game.events` 檔案內**。 另外一個 **`menu.events` 為遊戲主選單**,為有需要遊戲選單的人所新增的,若你不需要遊戲主畫面,可以參考下方修改方式。 **點進 `app.ts` 檔案**,找到如下方的程式碼,根據註解說明,**將字串後方的 `menu` 改成 `game` 即可**。 ```ts /** * 修改下方 source 的路徑字串,可以改變專案運行後初次執行的事件表 * 例如改為 'CG.VisualNovelGameTemplate/game.events' 可以直接執行遊戲 */ let source = 'CG.VisualNovelGameTemplate/menu.events'; // 請嘗試將這裡的 menu 改為 game ``` 至於該如何編輯 `menu.events` 來設計自己的遊戲主畫面,可以進入該檔案的「初始化」事件,找到「建立圖層佈局」的動作查看當前畫面佈局。 而對「建立圖層佈局」這個動作不熟悉的人,可以參考 **[【系統設計】CG 事件表的 UI 系統設計](https://www.youtube.com/live/BKRxSFObMlY?t=1000s)** 這個影片,簡單的介紹了如何使用該動作來建立自己的 UI 介面。 ## 教學資源 - 文章 - **[巴哈姆特 - 【教學】在網頁上做一個視覺小說遊戲](https://home.gamer.com.tw/artwork.php?sn=5866046)** - **[IT邦幫忙 - [Day 03] CG 同人陣的運作原理](https://ithelp.ithome.com.tw/articles/10321203)** - **[IT邦幫忙 - [Day 11] 在 CG 上公開自己的作品](https://ithelp.ithome.com.tw/articles/10328457)** - **[IT邦幫忙 - [Day 12] 設定專案封面、將成品發布到 Gamelet.online](https://ithelp.ithome.com.tw/articles/10328935)** - **[IT邦幫忙 - 什麼!在網頁上也可以寫視覺小說?](https://ithelp.ithome.com.tw/articles/10337454)** - 影片 - **[【遊戲設計】介紹在網頁上製作視覺小說遊戲](https://www.youtube.com/live/5AUGRityOec?t=189s)** - **[【系統設計】CG 事件表的 UI 系統設計](https://www.youtube.com/live/BKRxSFObMlY?t=1000s)** - **[【遊戲設計】視覺小說引擎更新報告!](https://www.youtube.com/live/s19SacUQ0V4?t=1092s)** - **[【教學】視覺小說引擎-迷霧特效【CC 字幕】](https://www.youtube.com/watch?v=MyXBfAL2BJQ)** - 本站討論串 - **[變數決定結局](https://code.gamelet.com/discuss/p/liaw1/issue/3698/0)** - **[分支選項設置](https://code.gamelet.com/discuss/coding/topic/3706)** ## 作者 **[cook1470](/profile/114899766849308759711@google)**
My Visual Novel
cook1470
visibility
code
OPEN
info_outline
# ## 開始設計 <a href="cg://source/CG.wawa123/game.events" class="mat-raised-button mat-primary">編輯遊戲事件表</a> <h2>遊戲介紹</h2> -- <strong>《這是一款異世界主題的視覺小說-小品遊戲》</strong> ...尚未完成...半殘品XD...因為沒時間做QQ.. 【立繪】**machi** 【劇本】 **machi** 【動畫】 **machi** 【ui小物件】 **machi** 【bgm】 【背景】 大多Ai魔法召喚 特別感謝 **酷可** 製作的視覺小說模組 -- ## 作者 **[machi](/profile/106963146897704619044@google)**
異世界餐廳經營
machi
visibility
code
OPEN
info_outline
# ntpugreenpolis 在這兒寫下一段簡短的文字介紹你的遊戲吧。 嗨,歡迎來到這,來玩一次奇異的冒險吧 ## 開始設計 <a href="cg://source/CG.ntpugreenpolis/game.events" class="mat-raised-button mat-primary">編輯遊戲事件表</a> ## 作者 **[Fan.Lin](/profile/108352734949056434584@google)**
ntpugreenpolis
Fan.Lin
visibility
code
OPEN
info_outline
# 造型測試 可以用這個專案測試你製作的造型。<br> 打開此專案後,按左上的餐刀叉複製此專案,<br> 複製後,按下右邊的資源管理,再按加載資源,隨便輸入一個代理名字,<br> 再按上傳資源,再按尋找檔案,選擇要測試的造型,然後按上傳資源,之後退出該頁面。<br> <br> 後續需要再看你現在在看的這一頁的話,這一頁是「README.md」,按它即可。<br> 上傳資源後,打開左邊的「CG.TWRole_Testing」資料夾,並按「TWRole_Testing.events」,<br> 把動作「角色外型」裏的角色換成自己要測試的角色,最後按試玩遊戲。<br> <br> 注意:若介意自己的造型出現在別人的專案裏,請在上傳資源時把「公開資源」關掉。<br> [光暈角色造型製作網頁](https://twrolecgeditor.gamelet.online/)
造型測試
Umeko
MORE RESULTS
ⒸCode.Gamelet.com |
Privacy Policy
|
Terms of Service